How to Resolve "Permission Denied" Errors in Kubernetes
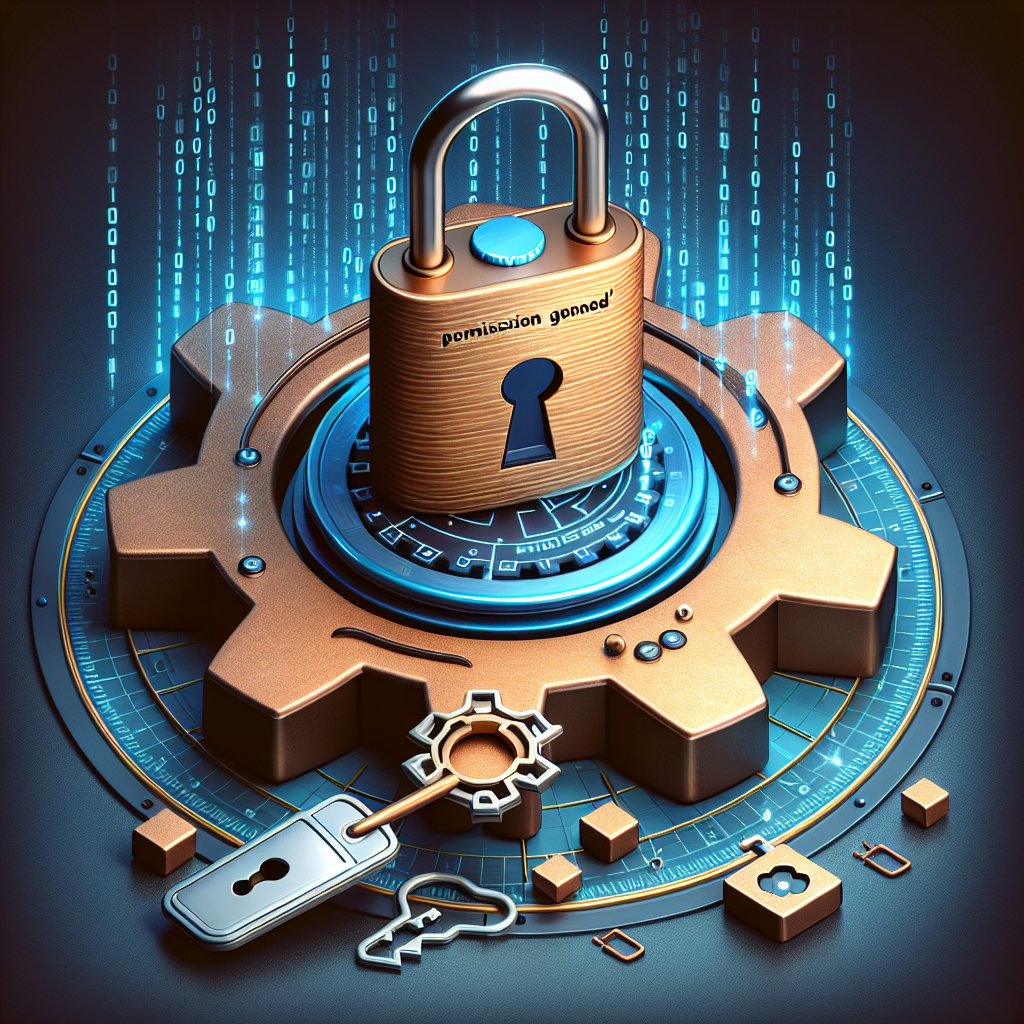
If you're working with Kubernetes, one error you might encounter is the "permission denied" error. This error can occur due to several reasons and often indicates that your application lacks the necessary permissions to perform a certain action or access certain resources.
Understanding the "Permission Denied" Error
The "permission denied" error may appear in various contexts, whether in logs, during container operations, or while attempting to access specific files or directories within a pod. This error essentially means that the user or service account running the application doesn’t have the required permissions.
Diagnosing the Issue
To diagnose the "permission denied" error, you should start by inspecting the logs for the affected pod:
kubectl logs <pod-name>
Look for any messages that suggest permission issues. For example, you might see messages like "permission denied" or "EACCES".
Common Causes and Solutions
1. File System Permissions
If your application is trying to access a file or directory, ensure that the permissions are correctly set. You might need to adjust the permissions within your Dockerfile or Kubernetes configuration. For instance, you can set permissions in your Dockerfile using:
RUN chown -R 1000:1000 /app
USER 1000
Additionally, you can ensure that volume mounts have the appropriate permissions set using an initContainer
:
initContainers:
- name: fix-permissions
image: busybox
command: ['sh', '-c', 'chown -R 1000:1000 /data']
volumeMounts:
- mountPath: /data
name: my-volume
2. Service Account Permissions
Kubernetes uses service accounts to manage pod permissions. If your pod needs specific permissions, it may require a Role or ClusterRole binding:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
Then, bind the role to the service account:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods
namespace: default
subjects:
- kind: ServiceAccount
name: default
namespace: default
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
3. Linux Capabilities
Sometimes, the pod might require additional Linux capabilities to perform certain actions. You can add these capabilities through the securityContext
field in your pod specification:
securityContext:
capabilities:
add: ["NET_ADMIN", "SYS_TIME"]
4. Network Policies
Network policies might restrict pod communication within the cluster. Ensure that your pod is permitted to communicate with the required services:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-app
spec:
podSelector:
matchLabels:
role: app
ingress:
- from:
- podSelector:
matchLabels:
role: db
Conclusion
Encountering the "permission denied" error in Kubernetes can disrupt your workflows and deployments. However, by systematically diagnosing and addressing the common causes outlined above, you can resolve these permission issues and ensure your applications run smoothly. Whether it's file system permissions, service account roles, Linux capabilities, or network policies, understanding the underlying cause will help you apply the correct fix.