Streamlining Kubernetes Deployments: How to Create and Deploy a Helm Chart for a Node.js Application
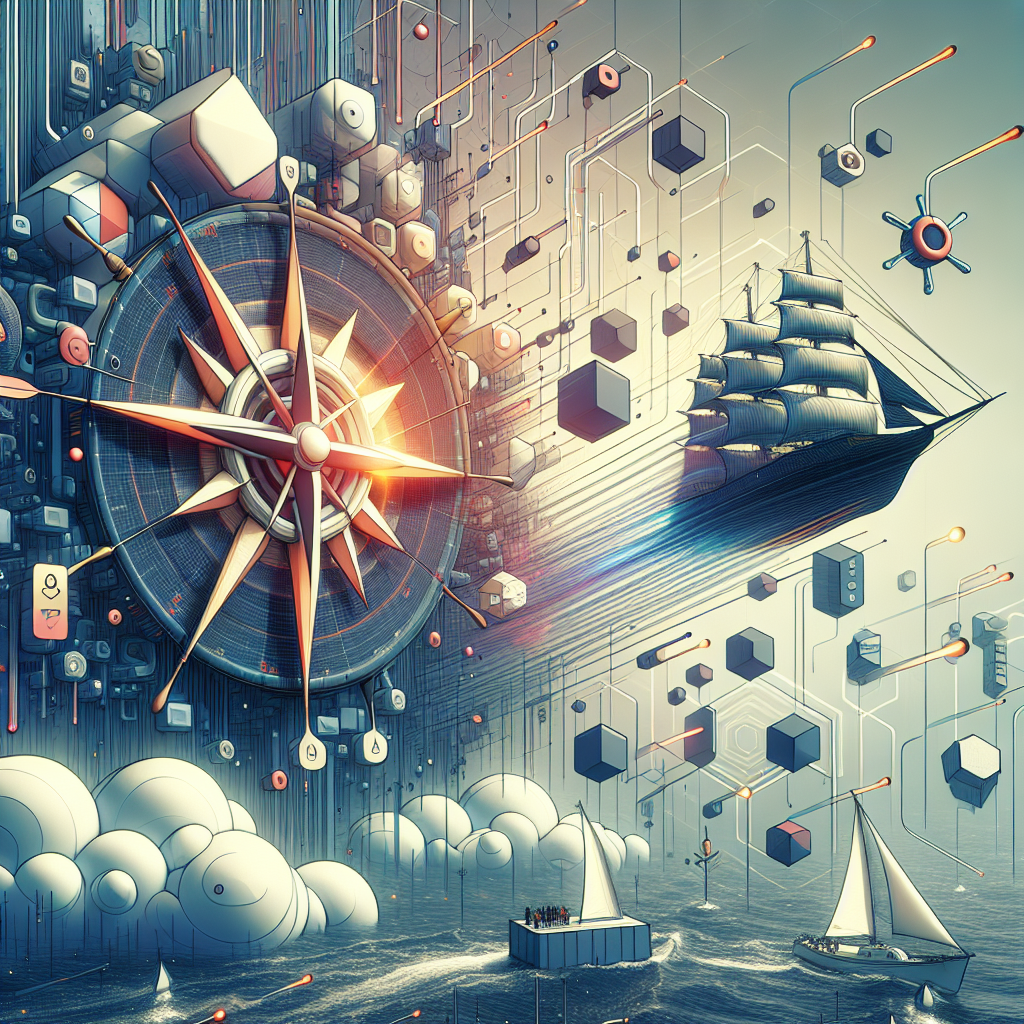
In the rapidly evolving landscape of cloud-native technologies, one tool that has gained significant traction for managing an application's lifecycle is Helm. Helm is often referred to as the package manager for Kubernetes, and it simplifies the deployment and management of Kubernetes applications through reusable, configurable packages called charts. In this blog post, we’ll walk through how to create and deploy a Helm chart for a simple Node.js application. We’ll also touch upon some advanced features of Helm and share lessons learned from real-world deployments.
Why Use Helm?
Helm offers several advantages:
- Simplifies Application Deployment: Helm charts enable easy deployment and management of Kubernetes applications.
- Reusability: Helm charts can be used across multiple environments and clusters, promoting consistency.
- Configuration Management: Helm makes it easy to manage application configurations through values files.
- Versioning: Helm charts support versioning, allowing you to roll back to previous versions effortlessly.
Prerequisites
Before you start, make sure you have the following:
- A Kubernetes cluster (This example uses Minikube for simplicity)
- Helm installed (you can follow the installation guide here)
- A basic understanding of Kubernetes concepts
Step 1: Create a Simple Node.js Application
First, we need a simple Node.js application to work with. Create a directory and initialize a Node.js project:
mkdir my-node-app
cd my-node-app
npm init -y
Create an app.js
file with the following content:
const http = require('http');
const PORT = process.env.PORT || 3000;
const requestHandler = (req, res) => {
res.end('Hello, from Node.js app!');
};
const server = http.createServer(requestHandler);
server.listen(PORT, (err) => {
if (err) {
return console.log('Error:', err);
}
console.log('Server is listening on', PORT);
});
Create a Dockerfile
to containerize the Node.js application:
FROM node:14
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "app.js"]
Build and push the Docker image to your preferred Docker registry:
# Build the Docker image
docker build -t your-docker-username/my-node-app .
# Push the Docker image to Docker Hub
docker push your-docker-username/my-node-app
Step 2: Create a Helm Chart
We'll use Helm to create a chart for our Node.js application. Create a Helm chart using the following command:
helm create my-node-app
This command will create a directory structure for your chart. Update the values.yaml
file to specify your Docker image:
# values.yaml
image:
repository: your-docker-username/my-node-app
tag: latest
pullPolicy: IfNotPresent
Update the templates/deployment.yaml
file to use the port 3000:
apiVersion: apps/v1
kind: Deployment
metadata:
name: {{ include "my-node-app.fullname" . }}
labels:
{{- include "my-node-app.labels" . | nindent 4 }}
spec:
replicas: {{ .Values.replicaCount }}
selector:
matchLabels:
{{- include "my-node-app.selectorLabels" . | nindent 6 }}
template:
metadata:
labels:
{{- include "my-node-app.selectorLabels" . | nindent 8 }}
spec:
containers:
- name: {{ .Chart.Name }}
image: "{{ .Values.image.repository }}:{{ .Values.image.tag }}"
imagePullPolicy: {{ .Values.image.pullPolicy }}
ports:
- containerPort: 3000
env:
- name: PORT
value: "3000"
Step 3: Deploy the Helm Chart
Deploy the Helm chart to your Kubernetes cluster:
helm install my-node-app ./my-node-app
Verify that the application is running:
kubectl get pods
kubectl get svc
Forward the port to access the application:
kubectl port-forward svc/my-node-app 8080:3000
Open your browser and navigate to http://localhost:8080. You should see Hello, from Node.js app!
Advanced Features of Helm
Helm offers several advanced features that provide more control and flexibility:
1. Rolling Back Releases
If you need to roll back to a previous release, use the following command:
helm rollback my-node-app 1
2. Managing Values and Configurations
You can override default chart values by providing a custom values.yaml
file or passing values directly from the command line:
# Using a custom values.yaml file
helm install my-node-app ./my-node-app -f custom-values.yaml
# Passing values via command line
helm install my-node-app ./my-node-app --set replicaCount=2
Lessons Learned and Common Pitfalls
Through real-world deployments, several lessons have been learned:
- Keep Charts Simple: Start with simple charts and gradually introduce complexity. Over-complicating charts can lead to maintenance challenges.
- Version Control: Make sure to version control your Helm charts along with your application's codebase for easy rollbacks and audits.
- Custom Values Management: Use separate values files for different environments (e.g., development, staging, production) to manage configurations effectively.
- Monitor Resource Usage: Ensure that your Kubernetes cluster has sufficient resources for both your applications and monitoring tools. Resource limits should be defined in your Helm charts.
Conclusion
Helm is an incredibly powerful tool for managing Kubernetes applications. By creating and deploying a Helm chart for a simple Node.js application, you can see how Helm simplifies the process and provides scalability. From managing configurations to rolling back releases, Helm addresses many challenges faced in cloud-native deployments. By following best practices and learning from real-world applications, you can leverage Helm to its full potential and streamline your Kubernetes operations.
Have you used Helm in your projects? Share your experiences, challenges, and tips in the comments below!